Introduction to Angular
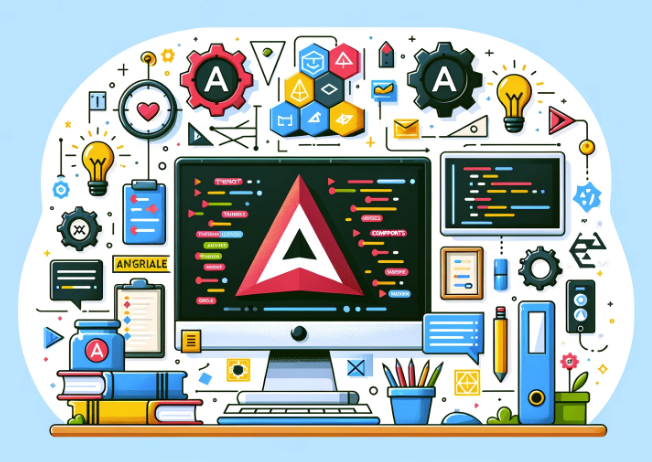
AI generated – Angular Overview
Table Of Contents
Importance of Angular
Angular is a platform and framework for building single-page client applications using HTML and TypeScript. Developed and maintained by Google, Angular provides a comprehensive solution for building dynamic and responsive web applications. It simplifies the development process by offering a robust set of tools and features, including a powerful CLI, dependency injection, and reactive programming support.
Key Features of Angular:
- Component-Based Architecture: Modular and reusable components.
- Two-Way Data Binding: Synchronizes data between the model and view.
- Dependency Injection: Efficiently manages service instances and dependencies.
- Reactive Programming: Built-in support for RxJS for reactive programming.
- Comprehensive CLI: Angular CLI for generating and managing projects.
- Built-in Routing: Powerful router for navigation and deep linking.
- Performance Optimization: Ahead-of-Time (AOT) compilation and lazy loading.
Uses of Angular
Angular is widely used across various domains, including:
- Enterprise Applications: Building scalable and maintainable business applications.
- Single-Page Applications (SPAs): Developing dynamic web applications with smooth navigation.
- E-commerce Platforms: Creating interactive and engaging online stores.
- Content Management Systems: Building flexible and customizable CMS solutions.
- Progressive Web Apps (PWAs): Developing offline-capable and responsive web applications.
Top-Tier Companies Using Angular
Several leading companies rely on Angular for their critical systems and applications:
- Google: Uses Angular for various internal and external projects.
- Microsoft: Employs Angular for some of its web applications.
- IBM: Utilizes Angular for building enterprise-level applications.
- Forbes: Uses Angular for its content management and delivery.
- Upwork: Employs Angular for its freelance platform interface.
Angular Learning Roadmap
Basic Level
- Introduction to Angular
- History and evolution of Angular
- Differences between AngularJS and Angular (2+)
- Setting up the development environment (Node.js, npm, Angular CLI)
- Creating Your First Angular Application
- Generating a new Angular project with Angular CLI
- Understanding the project structure
- Running the Angular application
- Basic Angular Concepts
- Modules and NgModule
- Components and templates
- Data binding (interpolation, property binding, event binding, two-way binding)
- Directives (structural and attribute directives)
- Services and dependency injection
- Angular Forms
- Template-driven forms
- Reactive forms
- Form validation
- Routing and Navigation
- Setting up routes
- Navigating between routes
- Route parameters and query parameters
- Lazy loading modules
Intermediate Level
- Advanced Components
- Component lifecycle hooks
- Input and Output properties
- Content projection with ng-content
- Dynamic components
- Data Management
- Using HttpClient for HTTP requests
- Observables and RxJS
- Handling asynchronous data streams
- Interacting with RESTful APIs
- State Management
- Introduction to state management
- Using NgRx for state management
- Actions, reducers, selectors, and effects
- Angular Animations
- Introduction to Angular animations
- Using the Angular animations library
- Creating and triggering animations
- Testing in Angular
- Unit testing with Jasmine and Karma
- Component testing with TestBed
- End-to-end testing with Protractor
- Writing effective test cases
Advanced Level
- Performance Optimization
- Ahead-of-Time (AOT) compilation
- Lazy loading and preloading modules
- Change detection strategies
- Optimizing Angular applications for performance
- Advanced Routing Techniques
- Nested routes and child routes
- Route guards (CanActivate, CanDeactivate)
- Custom route strategies
- Advanced lazy loading techniques
- Security in Angular
- Best practices for securing Angular applications
- Preventing XSS (Cross-Site Scripting) attacks
- Authentication and authorization strategies
- Implementing JWT (JSON Web Token) authentication
- Progressive Web Apps (PWAs)
- Introduction to PWAs
- Making Angular applications offline-capable
- Using service workers
- Implementing push notifications
- Angular Universal (Server-Side Rendering)
- Introduction to Angular Universal
- Setting up server-side rendering
- Benefits and use cases of SSR
- SEO optimization with Angular Universal
- Internationalization and Localization
- Setting up Angular applications for multiple languages
- Using Angular i18n tools
- Managing translations
- Dynamic language switching
- Advanced Development Techniques
- Custom pipes
- Advanced directives
- Custom form controls
- Creating reusable libraries
1. Introduction to Angular
- What is Angular?
- Overview of Angular as a front-end web framework developed by Google.
- Key features: Two-way data binding, Dependency Injection, Component-based architecture.
2. Setting Up Angular
- Installation and Setup
- Step-by-step guide to installing Angular CLI.
- Creating a new Angular project.
- Running the Angular development server.
JavaScript
npm install -g @angular/cli
ng new skiing-app
cd skiing-app
ng serve
3. Angular Architecture
- Modules and Components
- Explanation of NgModules and how they organize an Angular app.
- Overview of components, templates, and metadata.
Example: Creating a Skiing Component
JavaScript
// skiing.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-skiing',
templateUrl: './skiing.component.html',
styleUrls: ['./skiing.component.css']
})
export class SkiingComponent {
title = 'Skiing Adventures';
}
HTML