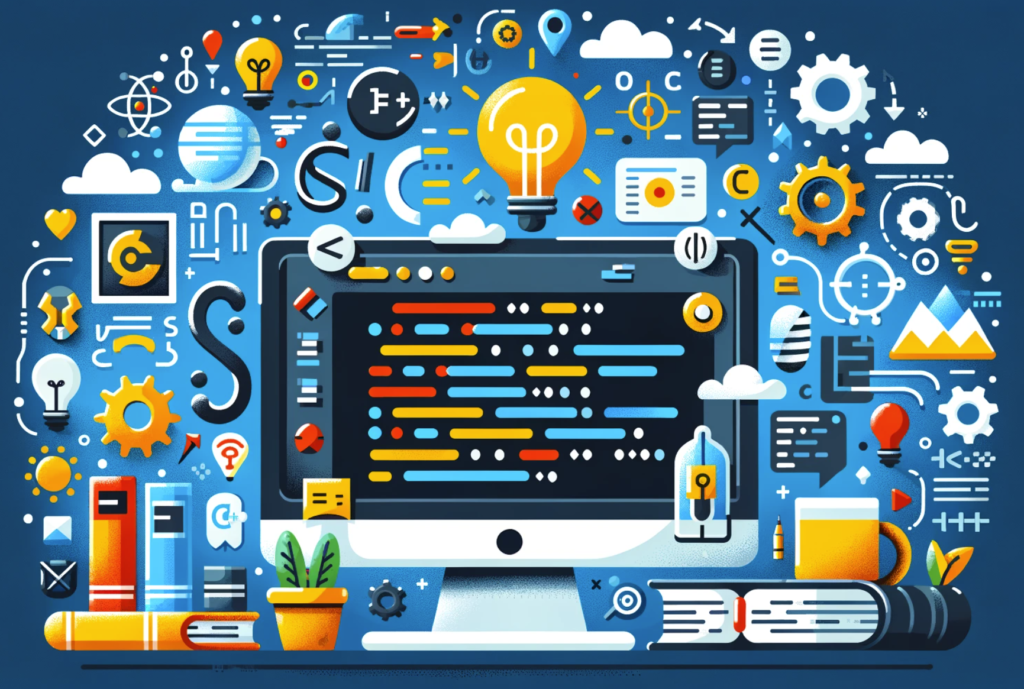
AI generated image for CSharp Roadmap
Introduction to C#
Importance of C#
C# (pronounced “C-Sharp”) is a modern, object-oriented programming language developed by Microsoft as part of its .NET initiative. C# is known for its versatility, robustness, and the ability to build a wide range of applications, from desktop to web and mobile applications. It combines the power and flexibility of C++ with the simplicity and productivity of Visual Basic.
Key Features of C#:
- Object-Oriented: Supports concepts like encapsulation, inheritance, and polymorphism.
- Component-Oriented: Facilitates building reusable, modular components.
- Type-Safe: Provides strong type checking to prevent type errors.
- Interoperability: Can interact with other languages and libraries, especially within the .NET ecosystem.
- Memory Management: Automatic garbage collection.
- Rich Standard Library: Extensive set of libraries for various tasks, including data structures, I/O, networking, and more.
- LINQ: Language Integrated Query for data querying capabilities.
Uses of C#
C# is used in a variety of domains, including:
- Desktop Applications: Windows Forms, WPF (Windows Presentation Foundation).
- Web Applications: ASP.NET for creating dynamic websites and services.
- Mobile Applications: Xamarin for cross-platform mobile development.
- Game Development: Unity game engine uses C# as its primary scripting language.
- Cloud Services: Azure cloud services development.
- Enterprise Software: Robust, scalable enterprise applications.
Top-Tier Companies Using C#
Several leading companies rely on C# for their critical systems and applications:
- Microsoft: Uses C# extensively in various products like Windows, Office, and Visual Studio.
- Stack Overflow: Uses C# for its web platform.
- Intuit: Uses C# for financial and business software.
- Siemens: Uses C# for industrial automation software.
- LinkedIn: Uses C# for some backend services.
- Accenture: Utilizes C# in various enterprise solutions.
C# Learning Roadmap
The roadmap is divided into three main levels: Basic, Intermediate, and Advanced. Each level builds on the previous one, ensuring a comprehensive understanding of C# programming.
Basic Level
- Introduction to C#
- History and evolution of C#
- Setting up the development environment (Installing .NET SDK, IDEs like Visual Studio, Visual Studio Code)
- Basic Syntax and Constructs
- Variables and Data Types
- Operators and Expressions
- Control Structures (if-else, switch, loops)
- Object-Oriented Programming (OOP)
- Classes and Objects
- Methods
- Constructors
- Inheritance
- Polymorphism
- Encapsulation
- Basic I/O
- Reading from and writing to the console
- Working with files (FileStream, StreamReader, StreamWriter)
- Exception Handling
- try-catch blocks
- Throwing exceptions
- Custom exceptions
- Collections Framework
- Lists (List, LinkedList)
- Sets (HashSet, SortedSet)
- Dictionaries (Dictionary, SortedDictionary)
- Iterators
Intermediate Level
- Advanced OOP Concepts
- Interfaces and Abstract Classes
- Delegates and Events
- Enums and Structs
- Properties and Indexers
- Generics
- Generic Classes and Methods
- Bounded Type Parameters
- Constraints on Generics
- Multithreading and Concurrency
- Thread lifecycle
- Creating and managing threads (Thread class, Task Parallel Library)
- Synchronization (lock, Monitor, Mutex)
- Concurrency utilities (Concurrent Collections, Parallel LINQ)
- LINQ (Language Integrated Query)
- Query syntax and method syntax
- LINQ to Objects
- LINQ to XML
- LINQ to SQL
- File I/O and Serialization
- Advanced file handling (FileStream, MemoryStream)
- XML and JSON serialization (System.Xml, System.Text.Json, Newtonsoft.Json)
- Networking
- Sockets and TCP/IP communication
- HTTP requests and responses (HttpClient)
- Database Interaction
- ADO.NET for database connectivity
- Executing SQL queries
- ORM with Entity Framework
Advanced Level
- Design Patterns
- Creational (Singleton, Factory)
- Structural (Adapter, Decorator)
- Behavioral (Observer, Strategy)
- C# Memory Management
- Garbage Collection
- Memory Leaks
- Profiling and Monitoring Tools (dotMemory, Visual Studio Profiler)
- Advanced Multithreading
- Asynchronous programming with async and await
- Task-based Asynchronous Pattern (TAP)
- Parallel Programming (Parallel class, PLINQ)
- ASP.NET Core
- Building web applications and APIs
- Middleware and Routing
- Dependency Injection
- Entity Framework Core for database interaction
- Xamarin for Mobile Development
- Building cross-platform mobile apps
- Xamarin.Forms for shared UI
- Integrating with platform-specific features
- Blazor for Web Development
- Building interactive web UIs with C#
- Blazor Server vs Blazor WebAssembly
- Security
- Authentication and Authorization (ASP.NET Identity)
- Secure coding practices
- Data encryption and decryption (System.Security.Cryptography)
- Testing
- Unit Testing with MSTest, NUnit, and xUnit
- Mocking with Moq
- Integration Testing
- Test-Driven Development (TDD)
Conclusion
Learning C# is a journey that starts with understanding the basics and gradually moves towards mastering advanced concepts and technologies. This roadmap is designed to guide you through this journey, ensuring you build a solid foundation before tackling more complex topics. By following this roadmap, you’ll gain the skills needed to develop robust, scalable, and high-performance applications using C#.
C#’s versatility and widespread adoption make it an essential skill for developers in various domains. Whether you’re just starting or looking to deepen your existing knowledge, this roadmap provides a comprehensive path to becoming proficient in C#.