Hibernate Overview
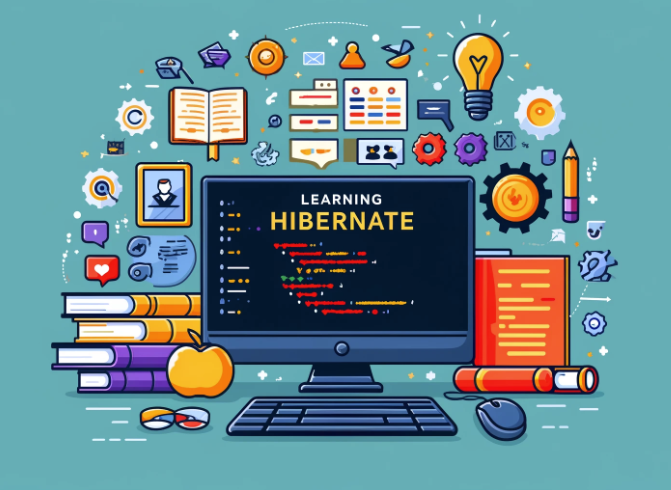
AI generated image for Learning Hibernate
Introduction to Hibernate
Importance of Hibernate
Hibernate is a popular open-source Object-Relational Mapping (ORM) framework for Java. It provides a framework for mapping an object-oriented domain model to a relational database. Hibernate simplifies the development of Java applications to interact with the database, offering a robust, high-performance, and scalable solution.
Key Features of Hibernate:
- Object-Relational Mapping: Maps Java objects to database tables and vice versa.
- HQL (Hibernate Query Language): Powerful, database-independent query language.
- Automatic Table Generation: Automatically generates database tables based on the entity mappings.
- Transaction Management: Manages transactions and concurrency control.
- Caching: Provides first-level and second-level caching to improve performance.
- Lazy Loading: Loads related objects on demand to enhance performance.
- Integration: Integrates seamlessly with Java EE, Spring, and other frameworks.
Uses of Hibernate
Hibernate is widely used across various domains, including:
- Enterprise Applications: Managing complex data relationships and transactions.
- E-commerce Platforms: Handling product catalogs, user data, and transactions.
- Banking and Finance: Managing financial records and transactional data.
- Healthcare Systems: Handling patient records and billing information.
- Government Systems: Managing large datasets with complex relationships.
Top-Tier Companies Using Hibernate
Several leading companies rely on Hibernate for their critical systems and applications:
- Red Hat: Uses Hibernate in various JBoss projects.
- LinkedIn: Employs Hibernate for data management.
- Amazon: Utilizes Hibernate for various backend services.
- IBM: Integrates Hibernate in some of its Java-based solutions.
- Oracle: Uses Hibernate as part of its middleware solutions.
Hibernate Learning Roadmap
Basic Level
- Introduction to Hibernate
- History and evolution of Hibernate
- Differences between Hibernate and other ORM frameworks
- Setting up the development environment (IDE setup, Maven/Gradle dependencies)
- Basic Configuration
- Hibernate configuration files (hibernate.cfg.xml)
- Setting up database connection properties
- Creating a simple Hibernate application
- Entity Mapping
- Mapping Java classes to database tables
- Using annotations for mapping (@Entity, @Table, @Id, @Column)
- Basic CRUD operations (Create, Read, Update, Delete)
- Hibernate Query Language (HQL)
- Introduction to HQL
- Writing basic HQL queries
- Using named queries
- Session and Transaction Management
- Understanding Session and SessionFactory
- Managing transactions (begin, commit, rollback)
- Using try-with-resources for session management
Intermediate Level
- Advanced Entity Mapping
- Mapping associations (one-to-one, one-to-many, many-to-one, many-to-many)
- Collection mappings (List, Set, Map, Bag)
- Component mappings (Embeddable types)
- Advanced HQL and Criteria API
- Writing complex HQL queries (joins, subqueries, aggregates)
- Using Criteria API for type-safe queries
- Query caching and optimization
- Inheritance Mapping
- Single Table Inheritance
- Table per Class Inheritance
- Joined Table Inheritance
- Caching
- First-level (session) caching
- Second-level caching (using EHCache, Infinispan)
- Query cache
- Integrating Hibernate with Other Frameworks
- Using Hibernate with Spring Framework
- Integrating Hibernate with Java EE applications
- Using Hibernate in a microservices architecture
Advanced Level
- Performance Tuning and Optimization
- Profiling and monitoring Hibernate applications
- Batch processing and bulk operations
- Optimizing fetch strategies (lazy vs. eager loading)
- Transaction Management and Concurrency Control
- Understanding transaction isolation levels
- Optimistic and pessimistic locking
- Managing concurrent access to data
- Advanced Caching Strategies
- Configuring and using advanced caching strategies
- Implementing custom cache regions
- Managing cache invalidation and expiration
- Custom Types and User Types
- Creating custom Hibernate types
- Implementing UserType and CompositeUserType interfaces
- Audit and Logging
- Implementing auditing for entity changes
- Using Envers for entity versioning and auditing
- Configuring Hibernate logging
- Advanced Integrations
- Integrating Hibernate with Spring Boot
- Using Hibernate with JPA (Java Persistence API)
- Advanced database operations with Hibernate
- Testing and Best Practices
- Writing unit tests for Hibernate applications
- Using in-memory databases for testing (H2, HSQLDB)
- Best practices for Hibernate development
Detailed Topics
1. Introduction to Hibernate
What is Hibernate?
Hibernate is a powerful, high-performance Object-Relational Persistence and Query service for any Java application. It provides a framework that helps in mapping the domain object-oriented model to a relational database.
Advantages of Using Hibernate
- Simplifies complex database operations.
- Supports transparent persistence.
- Automatic table creation and schema updates.
- Caching mechanism to optimize performance.
Installation and Configuration
Step 1: Add Hibernate Dependencies
<!-- Maven Dependency for Hibernate -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.10.Final</version>
</dependency>
Step 2: Create Configuration File (hibernate.cfg.xml
)
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/sports_db</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<property name="hibernate.hbm2ddl.auto">update</property>
</session-factory>
</hibernate-configuration>
2. Core Concepts
Session and SessionFactory
- SessionFactory: A factory for
Session
instances. It is a thread-safe object created once during the application startup. - Session: A single-threaded, short-lived object representing a conversation between the application and the persistent store.
Example: Initializing SessionFactory and Session
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory();
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Transaction Management
Handling transactions in Hibernate ensures the integrity and consistency of data.
Example: Transaction Management
Transaction transaction = null;
try {
transaction = session.beginTransaction();
// Perform database operations
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
} finally {
session.close();
}
Query Language (HQL)
HQL is similar to SQL but operates on persistent objects rather than tables.
Example: Simple HQL Query
List<Athlete> athletes = session.createQuery("FROM Athlete", Athlete.class).list();
for (Athlete athlete : athletes) {
System.out.println(athlete.getName());
}
3. Mappings
Basic Mappings
Mapping simple attributes between Java objects and database columns.
Example: Entity Class
@Entity
@Table(name = "athlete")
public class Athlete {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "sport")
private String sport;
// Getters and Setters
}
Collection Mappings
Mapping collections like List
, Set
, Map
.
Example: One-to-Many Mapping
@Entity
@Table(name = "team")
public class Team {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@OneToMany(mappedBy = "team", cascade = CascadeType.ALL)
private Set<Athlete> athletes = new HashSet<>();
// Getters and Setters
}
Association Mappings
Example: Many-to-Many Mapping
@Entity
@Table(name = "athlete")
public class Athlete {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@ManyToMany(cascade = { CascadeType.ALL })
@JoinTable(
name = "athlete_event",
joinColumns = { @JoinColumn(name = "athlete_id") },
inverseJoinColumns = { @JoinColumn(name = "event_id") }
)
Set<Event> events = new HashSet<>();
// Getters and Setters
}
@Entity
@Table(name = "event")
public class Event {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@ManyToMany(mappedBy = "events")
private Set<Athlete> athletes = new HashSet<>();
// Getters and Setters
}
4. Advanced Features
Criteria API
An alternative to HQL for creating programmatic queries.
Example: Criteria Query
CriteriaBuilder cb = session.getCriteriaBuilder();
CriteriaQuery<Athlete> cr = cb.createQuery(Athlete.class);
Root<Athlete> root = cr.from(Athlete.class);
cr.select(root).where(cb.equal(root.get("sport"), "soccer"));
Query<Athlete> query = session.createQuery(cr);
List<Athlete> results = query.getResultList();
Caching
Improves performance by reducing the number of database hits.
Example: Second-Level Cache Configuration
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
Interceptors and Listeners
Used for handling events in the Hibernate lifecycle.
Example: Interceptor
public class MyInterceptor extends EmptyInterceptor {
@Override
public boolean onSave(Object entity, Serializable id, Object[] state, String[] propertyNames, Type[] types) {
if (entity instanceof Athlete) {
// Perform some action
}
return super.onSave(entity, id, state, propertyNames, types);
}
}
5. Performance Tuning
Batch Processing
Efficiently handles bulk operations.
Example: Batch Insert
for (int i = 0; i < athletes.size(); i++) {
session.save(athletes.get(i));
if (i % 50 == 0) {
session.flush();
session.clear();
}
}
Lazy Loading vs Eager Loading
- Lazy Loading: Fetches related entities on-demand.
- Eager Loading: Fetches related entities immediately.
Example: Lazy Loading
@OneToMany(fetch = FetchType.LAZY, mappedBy = "team")
private Set<Athlete> athletes;
Fetch Strategies
Determines how Hibernate retrieves associated entities.
Example: Fetch Mode
Criteria criteria = session.createCriteria(Team.class);
criteria.setFetchMode("athletes", FetchMode.JOIN);
Conclusion
Hibernate is an essential framework for Java developers, providing a powerful and flexible ORM solution for managing relational data. By following this roadmap, you can develop a solid understanding of Hibernate’s features and capabilities, enabling you to create efficient and scalable data-driven applications.
Hibernate’s ability to simplify database interactions and its seamless integration with other frameworks make it an essential skill for modern Java developers. Whether you’re just starting or looking to deepen your existing knowledge, this roadmap provides a comprehensive path to becoming proficient in Hibernate.