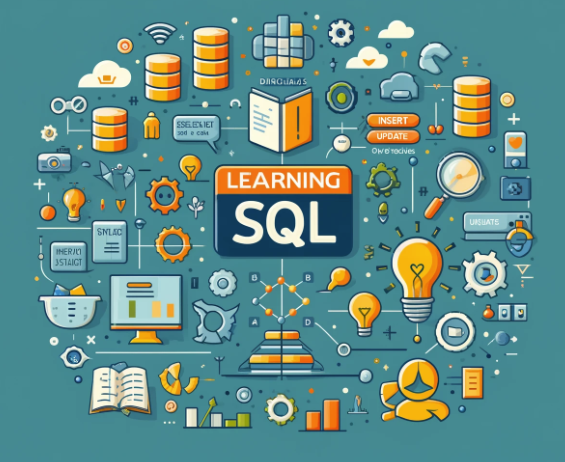
AI generated image for Learning SQL
SQL Overview
SQL (Structured Query Language) is a standardized language used to manage and manipulate relational databases. It is essential for tasks like querying, updating, and managing data. The roadmap to mastering SQL includes understanding its core concepts, commands, and advanced functionalities.
SQL Roadmap:
- Introduction to SQL
- Basic Concepts
- Database Models
- SQL Syntax and Structure
- Data Definition Language (DDL)
- CREATE
- ALTER
- DROP
- Data Manipulation Language (DML)
- SELECT
- INSERT
- UPDATE
- DELETE
- Data Control Language (DCL)
- GRANT
- REVOKE
- Transaction Control Language (TCL)
- COMMIT
- ROLLBACK
- SAVEPOINT
- SQL Functions and Aggregations
- Aggregate Functions (SUM, COUNT, AVG, etc.)
- Scalar Functions (UPPER, LOWER, etc.)
- Joins and Subqueries
- Inner Join
- Left Join
- Right Join
- Full Join
- Subqueries
- Advanced SQL Concepts
- Indexes
- Views
- Stored Procedures
- Triggers
- Performance Optimization
- Query Optimization
- Normalization
- Denormalization
- Practical Applications
- Case Studies
- Real-world Applications
Key SQL Concepts with Examples
1. Introduction to SQL
Concept: SQL is used to interact with databases, allowing users to execute commands to retrieve, update, and manage data. Understanding the basic syntax and structure is crucial for writing efficient SQL queries.
Example: Basic SQL Query
SELECT * FROM employees;
This query selects all columns from the ’employees’ table.
2. Data Definition Language (DDL)
Concept: DDL commands are used to define the structure of the database schema. They include commands like CREATE, ALTER, and DROP.
Example: Creating a Table
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
hire_date DATE,
department VARCHAR(50)
);
This command creates an ’employees’ table with specified columns.
3. Data Manipulation Language (DML)
Concept: DML commands are used to manipulate data within existing tables. These include SELECT, INSERT, UPDATE, and DELETE.
Example: Inserting Data
INSERT INTO employees (employee_id, first_name, last_name, hire_date, department)
VALUES (1, 'John', 'Doe', '2023-01-15', 'Engineering');
This command inserts a new row into the ’employees’ table.
4. Data Control Language (DCL)
Concept: DCL commands are used to control access to data within the database. They include GRANT and REVOKE.
Example: Granting Permissions
GRANT SELECT, INSERT ON employees TO 'username';
This command grants SELECT and INSERT permissions on the ’employees’ table to a specific user.
5. Transaction Control Language (TCL)
Concept: TCL commands manage transactions within the database. They include COMMIT, ROLLBACK, and SAVEPOINT.
Example: Transaction Management
BEGIN TRANSACTION;
INSERT INTO employees (employee_id, first_name, last_name, hire_date, department)
VALUES (2, 'Jane', 'Smith', '2023-02-20', 'Marketing');
COMMIT;
This sequence of commands starts a transaction, inserts a new row, and then commits the transaction.
6. SQL Functions and Aggregations
Concept: SQL functions perform operations on data, while aggregate functions summarize data.
Example: Aggregate Function
SELECT department, COUNT(*) AS num_employees
FROM employees
GROUP BY department;
This query counts the number of employees in each department.
7. Joins and Subqueries
Concept: Joins combine rows from two or more tables based on related columns. Subqueries are nested queries used within another SQL query.
Example: Inner Join
SELECT employees.first_name, employees.last_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department = departments.department_id;
This query joins ’employees’ and ‘departments’ tables to display employee names and their department names.
8. Advanced SQL Concepts
Concept: Advanced concepts include indexes for performance optimization, views for simplifying complex queries, and stored procedures for reusable logic.
Example: Creating an Index
CREATE INDEX idx_department ON employees (department);
This command creates an index on the ‘department’ column of the ’employees’ table to improve query performance.
9. Performance Optimization
Concept: Optimizing SQL queries involves strategies like indexing, query rewriting, and schema design techniques like normalization.
Example: Query Optimization
EXPLAIN SELECT * FROM employees WHERE department = 'Engineering';
This command uses the EXPLAIN keyword to analyze the query execution plan, helping identify potential performance bottlenecks.
10. Practical Applications
Concept: Applying SQL in real-world scenarios involves understanding how to structure data for business applications, data analysis, and reporting.
Example: Case Study
Consider an e-commerce database where SQL is used to track sales, inventory, and customer data. Queries can be written to generate sales reports, track inventory levels, and analyze customer behavior.