Microservices Overview
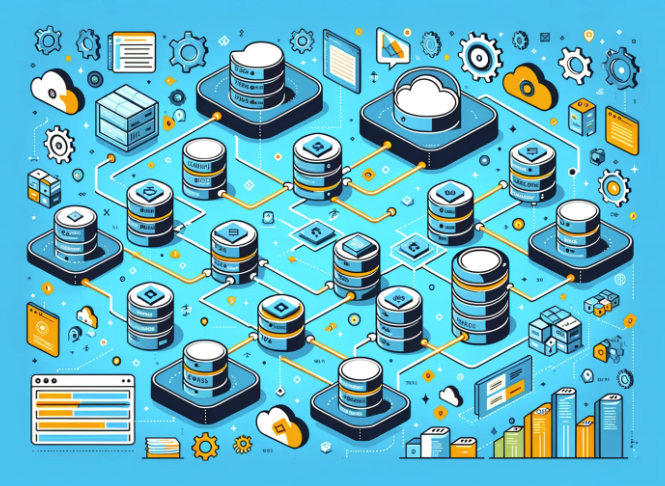
AI generated image for Microservices Roadmap
Importance of Microservices
Microservices is an architectural style that structures an application as a collection of small, autonomous services modeled around a business domain. Each microservice is independently deployable, scalable, and capable of communicating with others through APIs. This approach offers several advantages over traditional monolithic architectures, including enhanced modularity, flexibility, and ease of deployment.
Key Features of Microservices:
- Modularity: Breaks down applications into smaller, manageable pieces.
- Independent Deployment: Each service can be deployed independently.
- Scalability: Services can be scaled individually based on demand.
- Technology Diversity: Different services can use different technologies.
- Fault Isolation: Failure in one service does not impact others.
- Continuous Delivery: Simplifies continuous integration and delivery (CI/CD).
Uses of Microservices
Microservices are widely used across various domains, including:
- E-commerce Platforms: Managing catalog, cart, and order services.
- Financial Services: Handling customer accounts, transactions, and analytics.
- Healthcare Systems: Managing patient records, appointments, and billing.
- Media and Entertainment: Delivering streaming content and user recommendations.
- Social Media Platforms: Handling user profiles, messaging, and feeds.
Top-Tier Companies Using Microservices
Several leading companies rely on microservices for their critical systems and applications:
- Netflix: Uses microservices for its streaming service and recommendation engine.
- Amazon: Utilizes microservices for its e-commerce platform and AWS services.
- Spotify: Employs microservices for music streaming and user recommendations.
- Uber: Uses microservices for ride-hailing, payments, and analytics.
- Airbnb: Relies on microservices for its booking and property management systems.
Microservices Learning Roadmap
Basic Level
- Introduction to Microservices
- History and evolution of microservices
- Differences between monolithic and microservices architectures
- Benefits and challenges of microservices
- Microservices Fundamentals
- Key principles of microservices (single responsibility, decentralized data management)
- Basic concepts (service discovery, API gateway, circuit breaker)
- Setting up the development environment (Docker, Kubernetes, IDE setup)
- Building Your First Microservice
- Creating a simple microservice with Spring Boot or Node.js
- Understanding RESTful APIs
- Implementing basic CRUD operations
- Communication Between Microservices
- Synchronous communication with HTTP/REST
- Introduction to asynchronous communication with messaging (RabbitMQ, Kafka)
- Using service discovery (Eureka, Consul)
Intermediate Level
- Advanced Microservices Concepts
- Service registry and discovery
- API Gateway pattern (Zuul, API Gateway)
- Circuit Breaker pattern (Hystrix, Resilience4j)
- Load balancing (Ribbon, Spring Cloud LoadBalancer)
- Data Management in Microservices
- Database per service pattern
- Managing distributed transactions (sagas, two-phase commit)
- Event sourcing and CQRS (Command Query Responsibility Segregation)
- Microservices Security
- Implementing authentication and authorization (OAuth2, JWT)
- Securing inter-service communication (TLS, mTLS)
- Using security gateways
- Testing Microservices
- Unit testing with JUnit, Mockito
- Integration testing with Spring Boot Test, Testcontainers
- Contract testing with Pact
- End-to-end testing strategies
Advanced Level
- Microservices Orchestration and Choreography
- Understanding orchestration (BPMN, Camunda)
- Implementing choreography with event-driven architecture
- Saga pattern for distributed transactions
- Advanced Communication Patterns
- Using gRPC for efficient inter-service communication
- Implementing event-driven microservices with Kafka
- Handling eventual consistency and data synchronization
- Monitoring and Observability
- Implementing logging with ELK Stack (Elasticsearch, Logstash, Kibana)
- Monitoring with Prometheus and Grafana
- Distributed tracing with Zipkin or Jaeger
- Performance Optimization and Scalability
- Profiling and optimizing microservices
- Implementing caching strategies (Redis, Memcached)
- Auto-scaling with Kubernetes
- Deployment and DevOps
- Containerizing microservices with Docker
- Orchestrating microservices with Kubernetes
- Implementing CI/CD pipelines with Jenkins, GitLab CI, or GitHub Actions
- Blue-Green and Canary deployments
- Case Studies and Best Practices
- Analyzing real-world microservices architectures
- Best practices for microservices design and implementation
- Handling microservices anti-patterns
Deep Dive into Individual Topics
1. Introduction to Microservices
Definition:
Microservices architecture is an approach to software development where a large application is built as a suite of small services, each running its own process and communicating with lightweight mechanisms, often HTTP resources, or messaging queues.
Example:
Imagine a sports league management system for an extravagant sport like Quidditch. Each aspect of the system, such as team management, match scheduling, scoring, and fan engagement, is handled by separate microservices.
2. Core Concepts
Services:
Self-contained units that encapsulate a specific business capability. For example, a “Team Management Service” for handling team-related operations.
APIs:
Standardized interfaces through which services interact. RESTful APIs are commonly used.
Communication Protocols:
- HTTP/REST: Simple and widely used.
- gRPC: High-performance RPC framework.
- Messaging: RabbitMQ or Kafka for asynchronous communication.
3. Designing Microservices
Domain-Driven Design (DDD):
Focuses on the core domain and domain logic. Splitting the application based on business capabilities.
Example:
In our Quidditch system:
- Team Management Service
- Match Scheduling Service
- Scoring Service
- Fan Engagement Service
Identifying Microservices:
Analyze the domain and divide it into bounded contexts. Each context becomes a candidate for a microservice.
4. Microservices Patterns
Service Discovery:
Allows services to find each other dynamically.
Example:
Using Netflix Eureka for service registration and discovery in a Spring Boot application.
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Circuit Breaker:
Prevents cascading failures by stopping requests to a failing service.
Example:
Using Resilience4j for implementing a circuit breaker.
@Bean
public CircuitBreakerConfig customCircuitBreakerConfig() {
return CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.slidingWindowSize(2)
.build();
}
API Gateway:
A single entry point for all clients.
Example:
Implementing Zuul API Gateway in a Spring Boot application.
@SpringBootApplication
@EnableZuulProxy
public class ApiGatewayApplication {
public static void main(String[] args) {
SpringApplication.run(ApiGatewayApplication.class, args);
}
}
Event Sourcing:
Stores changes to the application state as a sequence of events.
Saga Pattern:
Manages distributed transactions.
5. Implementing Microservices with Java
Spring Boot:
A framework for building microservices.
Example:
Creating a simple “Team Management Service”.
@RestController
@RequestMapping("/teams")
public class TeamController {
@GetMapping("/{id}")
public Team getTeam(@PathVariable String id) {
// Implementation to retrieve team by ID
}
@PostMapping
public Team createTeam(@RequestBody Team team) {
// Implementation to create a new team
}
}
Spring Cloud:
Integrates Spring Boot with cloud solutions.
JHipster:
A development platform to generate, develop, and deploy Spring Boot + Angular/React/Vue web applications.6. Microservices Infrastructure
Containers and Orchestration:
- Docker: Containerization platform.
- Kubernetes: Orchestration platform for managing containerized applications.
Service Mesh:
- Istio: Provides secure, control, and observable communication between microservices.
7. Reliability and Fault-Tolerance
Resilience4j:
A lightweight fault tolerance library inspired by Netflix Hystrix.
Example:
Implementing a retry mechanism.
RetryConfig config = RetryConfig.custom()
.maxAttempts(3)
.waitDuration(Duration.ofMillis(500))
.build();
RetryRegistry registry = RetryRegistry.of(config);
Retry retry = registry.retry("teamService");
Supplier<Team> supplier = Retry.decorateSupplier(retry, () -> teamService.getTeamById("team1"));
Team team = supplier.get();
8. Service Availability and Discoverability
Eureka:
Service discovery and registration.
Example:
Registering a service with Eureka.
@SpringBootApplication
@EnableEurekaClient
public class TeamServiceApplication {
public static void main(String[] args) {
SpringApplication.run(TeamServiceApplication.class, args);
}
}
Consul:
A tool for service discovery and configuration.
9. Testing Microservices
Unit Testing:
Testing individual components.
Integration Testing:
Testing interactions between components.
Contract Testing:
Ensuring that services communicate as expected.
10. Deploying Microservices
CI/CD Pipelines:
Automating the deployment process.
Example:
Using Jenkins for continuous integration and deployment.
Kubernetes Deployments:
Managing deployments in Kubernetes.
Monitoring and Logging:
Using tools like Prometheus and Grafana for monitoring, and ELK stack for logging.
Conclusion
Microservices architecture provides a robust framework for building scalable, maintainable, and resilient applications. By following this roadmap, you can develop a solid understanding of microservices principles and practices, enabling you to design and implement effective microservices-based solutions.
Microservices’ modularity, independent deployment, and scalability make it an essential architecture for modern software development. Whether you’re just starting or looking to deepen your existing knowledge, this roadmap provides a comprehensive path to becoming proficient in microservices.