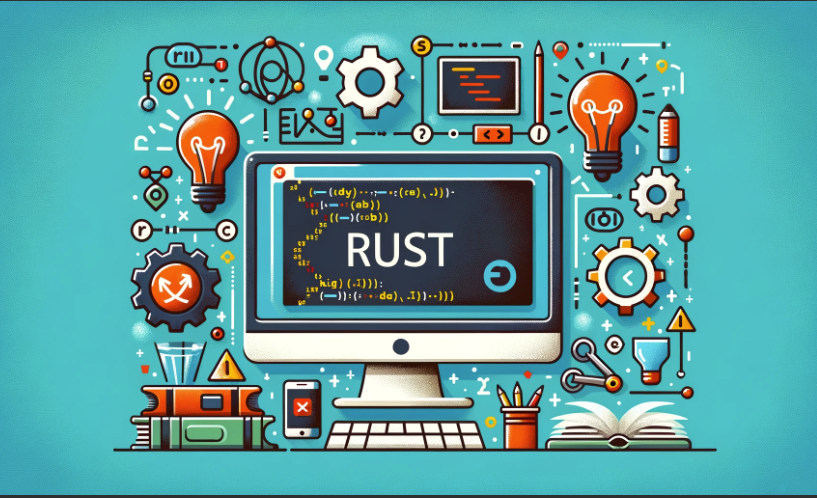
AI generated image for Programming in Rust
Introduction to Rust
Importance of Rust
Rust is a systems programming language that is designed for performance and safety, particularly safe concurrency. It aims to provide the control and performance of a low-level language while offering the safety guarantees and ergonomics of a high-level language. Rust’s unique ownership system ensures memory safety without needing a garbage collector, making it highly efficient.
Key Features of Rust:
- Memory Safety: Ownership system ensures memory safety without garbage collection.
- Performance: Comparable to C and C++ due to fine-grained control over system resources.
- Concurrency: Safe concurrency models prevent data races.
- Zero-Cost Abstractions: High-level abstractions compile down to low-level code without performance penalties.
- Type Safety: Strong static type system prevents many programming errors.
- Tooling: Integrated package manager (Cargo) and build system.
Uses of Rust
Rust is used in a variety of domains, including:
- Systems Programming: Operating systems, file systems, and other low-level tasks.
- Web Development: WebAssembly and backend services.
- Networking: High-performance network services.
- Embedded Systems: Programming microcontrollers and other hardware.
- Game Development: Performance-critical game engines and tools.
- Cryptography: Implementing secure systems and protocols.
Top-Tier Companies Using Rust
Several leading companies rely on Rust for their critical systems and applications:
- Mozilla: Rust was developed by Mozilla and is used in their projects, including the Servo browser engine.
- Dropbox: Uses Rust for performance-critical components.
- Microsoft: Uses Rust for secure and reliable systems.
- Amazon: Uses Rust for various internal tools and services.
- Facebook: Uses Rust for backend services and performance-sensitive tasks.
- Cloudflare: Uses Rust for network services and cryptographic operations.
Rust Learning Roadmap
The roadmap is divided into three main levels: Basic, Intermediate, and Advanced. Each level builds on the previous one, ensuring a comprehensive understanding of Rust programming.
Basic Level
- Introduction to Rust
- History and evolution of Rust
- Setting up the development environment (Installing Rust, using rustup, and IDEs like VSCode)
- Basic Syntax and Constructs
- Variables and Mutability
- Data Types
- Functions and Control Structures (if-else, loops, match)
- Ownership and Borrowing
- Ownership Rules
- References and Borrowing
- Slices
- Structs and Enums
- Defining and using Structs
- Defining and using Enums
- Pattern Matching
- Basic I/O
- Reading from and writing to the console
- Working with files (std::fs)
- Error Handling
- Result and Option types
- Error Propagation (using
?
operator) - Custom Error Types
- Collections
- Vectors
- Strings
- HashMaps
Intermediate Level
- Advanced Structs and Enums
- Tuple Structs
- Unit-Like Structs
- Enum Variants with Data
- Traits and Generics
- Defining and Implementing Traits
- Using Traits for Polymorphism
- Generics and Type Parameters
- Trait Bounds
- Lifetimes
- Understanding Lifetimes
- Lifetime Annotations
- Lifetime Elision
- Modules and Packages
- Creating and Using Modules
- The
mod
anduse
Keywords - Packages and Crates (using Cargo)
- Managing Dependencies
- Concurrency
- Threads (std::thread)
- Message Passing (std::sync::mpsc)
- Shared State Concurrency (Mutex, Arc)
- File I/O and Serialization
- Reading and Writing Files
- JSON and TOML Serialization (serde crate)
- Testing
- Writing Tests in Rust
- Using Cargo to Run Tests
- Test-Driven Development (TDD)
Advanced Level
- Advanced Traits
- Trait Objects
- Dynamic Dispatch
- Operator Overloading
- Memory Management
- Smart Pointers (Box, Rc, Arc)
- Interior Mutability (RefCell, Cell)
- Asynchronous Programming
- Async-Await Syntax
- Futures and Executors
- Using the async-std and tokio crates
- Web Development
- Building Web Applications with Actix and Rocket
- RESTful APIs with Warp
- WebAssembly (wasm-bindgen, stdweb)
- Systems Programming
- Low-Level Programming with Rust
- Writing Safe and Efficient Code
- Using the libc and winapi Crates
- Embedded Systems
- Programming Microcontrollers with Rust
- Using the
embedded-hal
crate - Real-Time Operating Systems (RTOS) in Rust
- Game Development
- Using the Amethyst and Bevy Engines
- Performance Optimization
- Interfacing with C Libraries
- Networking
- Building High-Performance Network Servers with Tokio
- Asynchronous Networking
- Protocol Implementation
- Security
- Secure Coding Practices
- Cryptography with Rust (using the rust-crypto crate)
- Safe Rust and Unsafe Rust
Conclusion
Learning Rust is a journey that starts with understanding the basics and gradually moves towards mastering advanced concepts and technologies. This roadmap is designed to guide you through this journey, ensuring you build a solid foundation before tackling more complex topics. By following this roadmap, you’ll gain the skills needed to develop robust, safe, and high-performance applications using Rust.
Rust’s focus on safety, performance, and concurrency makes it an essential skill for developers in various domains. Whether you’re just starting or looking to deepen your existing knowledge, this roadmap provides a comprehensive path to becoming proficient in Rust.